728x90
반응형
controller단에서 매개변수를 넘겨받는 방법을 한번 정리하고 넘어가고 싶어 포스팅을 남기게 되었다.
1. /test/{id} 로 넘겨받고 싶은 경우
- @PathVariable
/* /test/{id} */
@GetMapping("/test/{id}")
public String testControllerWithPathVariables(@PathVariable(required = false) int id){
return "hello id : " + id;
}
2. /test?id=123으로 넘겨받고 싶은 경우
- @RequestParam
/* /test/testRequestParam?id=123 */
@GetMapping("/test/testRequestParam")
public String testControllerWithPathVariables(@RequestParam(required = false) int id){
return "hello id : " + id;
}
3. 객체를 넘겨받고 싶은 경우
- @RequestBody
- 복잡한 자료형을 넘겨받고 싶은 경우를 말한다.
다양한 자료형을 가진 편지 객체.
@Data
public class LetterDTO {
private String id;
private String title; // 편지 제목
private String content; // 편지 내용
private LocalDateTime receivedDate; // 받을 날짜 지정
private String receivedPhoneNumber; // 받을 사람 휴대폰 번호 지정
}
@GetMapping("/test/testRequestBody")
public String testControllerWithPathVariables(@RequestBody(required = false) LetterDTO dto){
return "hello id : " + dto.getId() + "hello content : " + dto.getContent();
}
4. 객체를 리턴하고 싶을 경우
- 복잡한 자료형을 반환하고싶을 경우에는 어떻게 해야할까?
- @RestController를 알아보자
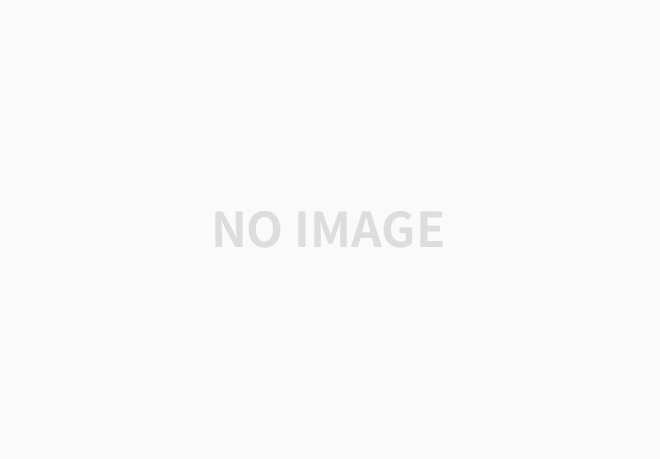
- @Controller
: @Component이다. 스프링이 이 클래스의 오브젝트를 알아서 생성하고, 다른 오브젝트들과의 의존성을 연결한다는 뜻이다.
- @ResponseBody
: 이 클래스가 리턴하는 것은 웹 서비스의 ResponseBody라는 뜻이다.
: 메서드 리턴 시 스프링은 리턴된 객체를 JSON으로 바꾸고 이를 HttpResponse에 담아 반환한다.
- 다양한 DTO를 리턴하기위해 API의 리턴타입은 ResponseEntity<T> 로 통일하는 것을 권장한다.
: 헤더와 HTTP Status를 조작할 수 있다.
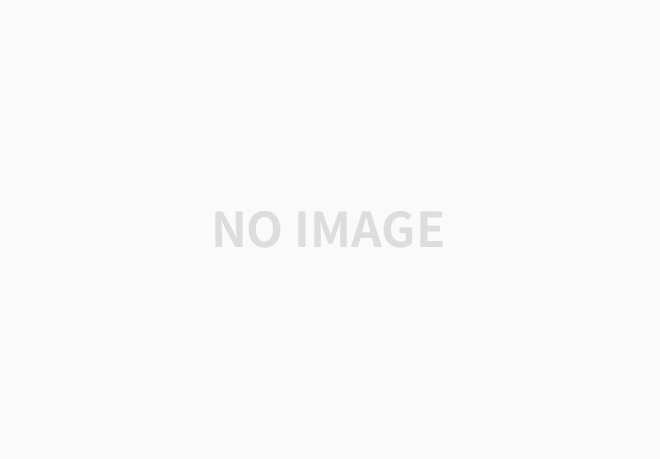
5. 직렬화(Serialization)
- 오브젝트를 저장하거나 네트워크를 통해 전달할 수 있는 형태로 변환하는 것
- 역직렬화 : 전환된 형태를 오브젝트로 저장하는 것
728x90
반응형
'Dev > SpringBoot' 카테고리의 다른 글
JPA 사용해서 무한 계층 댓글 구현해보기 - 03 (0) | 2022.01.20 |
---|---|
JPA 사용해서 무한 계층 댓글 구현해보기 - 02 (0) | 2022.01.20 |
JPA 사용해서 무한 계층 댓글 구현해보기 - 01 (0) | 2022.01.20 |
34. [JPA] 02. JPA 시작 (0) | 2021.11.07 |
33. [JPA] 01. JPA 소개 (0) | 2021.11.07 |