반응형
문제
10. 미로탐색(DFS)
설명
7*7 격자판 미로를 탈출하는 경로의 가지수를 출력하는 프로그램을 작성하세요.
출발점은 격자의 (1, 1) 좌표이고, 탈출 도착점은 (7, 7)좌표이다. 격자판의 1은 벽이고, 0은 통로이다.
격자판의 움직임은 상하좌우로만 움직인다. 미로가 다음과 같다면
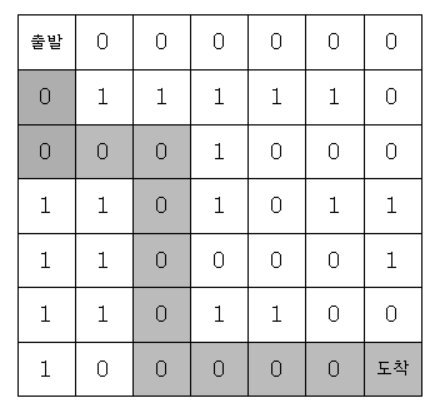
위의 지도에서 출발점에서 도착점까지 갈 수 있는 방법의 수는 8가지이다.
입력
7*7 격자판의 정보가 주어집니다.
출력
첫 번째 줄에 경로의 가지수를 출력한다.
예시 입력 1
0 0 0 0 0 0 0
0 1 1 1 1 1 0
0 0 0 1 0 0 0
1 1 0 1 0 1 1
1 1 0 0 0 0 1
1 1 0 1 1 0 0
1 0 0 0 0 0 0
예시 출력 1
8
코드
import java.util.Scanner;
class Main {
static int [][] map;
static boolean [][] check;
static int n,m;
// 하 우 상 좌
static int [] nx = {1,0,-1,0};
static int [] ny = {0,1,0,-1};
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
n = 0;
map = new int[8][8];
check = new boolean[8][8];
for (int i = 1; i < 8; i++) {
for (int j = 1; j < 8; j++) {
map[i][j] = sc.nextInt();
}
}
// 초기화 하는게 중요
check[1][1] = true;
dfs(1,1);
System.out.println(n);
}
private static void dfs(int a, int b) {
if(a==7 && b==7){
n++;
}else{
for (int i = 0; i < 4; i++) {
int nextX = a+nx[i];
int nextY = b+ny[i];
if((0< nextX && nextX <8 && 0< nextY && nextY < 8) && !check[nextX][nextY] && map[nextX][nextY] == 0){
check[nextX][nextY] = true;
dfs(nextX,nextY);
check[nextX][nextY] = false;
}
}
}
}
}
반응형
'알고리즘 > DFS, BFS, 시뮬, 백트래킹' 카테고리의 다른 글
[백준] 2578번 빙고 #Java (0) | 2022.05.25 |
---|---|
[백준] 2630번 색종이 만들기 (0) | 2022.05.17 |
[프로그래머스] N-Queen 문제 (0) | 2021.11.09 |
[프로그래머스] 타겟 넘버 - BFS (0) | 2021.09.20 |
[백준] 단지번호 붙이기 - BFS (0) | 2021.09.20 |